728x90
CubeMap.cpp, h (Framework)
더보기
#include "Framework.h"
#include "CubeMap.h"
CubeMap::CubeMap(Shader * shader)
:shader(shader)
{
mesh = new MeshCube(shader);
//mesh = new MeshSphere(shader, 0.5f, 20 , 20);
sSrv = shader->AsSRV("CubeMap");
}
CubeMap::~CubeMap()
{
SafeDelete(mesh);
SafeRelease(srv);
}
void CubeMap::Texture(wstring file)
{
SafeRelease(srv);
file = L"../../_Textures/" + file;
Check(D3DX11CreateShaderResourceViewFromFile(
D3D::GetDevice(), file.c_str(), NULL, NULL, &srv , NULL
));
}
void CubeMap::Update()
{
mesh->Update();
}
void CubeMap::Render()
{
sSrv->SetResource(srv);
mesh->Render();
}
void CubeMap::Pass(UINT val)
{
mesh->Pass(val);
}
void CubeMap::Position(float x, float y, float z)
{
mesh->Position(x, y, z);
}
void CubeMap::Position(Vector3 & vec)
{
mesh->Position(vec);
}
void CubeMap::Position(Vector3 * vec)
{
mesh->Position(vec);
}
void CubeMap::Rotation(float x, float y, float z)
{
mesh->Rotation(x, y, z);
}
void CubeMap::Rotation(Vector3 & vec)
{
mesh->Rotation(vec);
}
void CubeMap::Rotation(Vector3 * vec)
{
mesh->Rotation(vec);
}
void CubeMap::RotationDegree(float x, float y, float z)
{
mesh->RotationDegree(x, y, z);
}
void CubeMap::RotationDegree(Vector3 & vec)
{
mesh->RotationDegree(vec);
}
void CubeMap::RotationDegree(Vector3 * vec)
{
mesh->RotationDegree(vec);
}
void CubeMap::Scale(float x, float y, float z)
{
mesh->Scale(x, y, z);
}
void CubeMap::Scale(Vector3 & vec)
{
mesh->Scale(vec);
}
void CubeMap::Scale(Vector3 * vec)
{
mesh->Scale(vec);
}
#pragma once
class CubeMap
{
public:
CubeMap(Shader* shader);
~CubeMap();
void Texture(wstring file);
void Update();
void Render();
public:
void Pass(UINT val);
void Position(float x, float y, float z);
void Position(Vector3& vec);
void Position(Vector3* vec);
void Rotation(float x, float y, float z);
void Rotation(Vector3& vec);
void Rotation(Vector3* vec);
void RotationDegree(float x, float y, float z);
void RotationDegree(Vector3& vec);
void RotationDegree(Vector3* vec);
void Scale(float x, float y, float z);
void Scale(Vector3& vec);
void Scale(Vector3* vec);
private:
Shader* shader;
UINT pass = 0;
Mesh* mesh;
ID3D11ShaderResourceView* srv = NULL;
ID3DX11EffectShaderResourceVariable* sSrv;
};
CubeMapDemo.cpp, h (UnitTest)
더보기
#include "stdafx.h"
#include "CubeMapDemo.h"
void CubeMapDemo::Initialize()
{
Context::Get()->GetCamera()->RotationDegree(25, 0, 0);
Context::Get()->GetCamera()->Position(1, 36, -85);
((Freedom*)Context::Get()->GetCamera())->Speed(50);
shader = new Shader(L"15_Mesh.fxo");
CreateMesh();
sDirection = shader->AsVector("LightDirection");
cubeMapShader = new Shader(L"16_CubeMap.fxo");
cubeMap = new CubeMap(cubeMapShader);
cubeMap->Texture(L"Environment/DesertCube1024.dds");
cubeMap->Position(0, 20, 0);
cubeMap->Scale(10, 10, 10);
}
void CubeMapDemo::Destroy()
{
SafeDelete(shader);
SafeDelete(quad);
SafeDelete(plane);
SafeDelete(cube);
for (UINT i = 0; i < 10; i++)
{
SafeDelete(cylinder[i]);
SafeDelete(sphere[i]);
}
SafeDelete(cubeMapShader);
SafeDelete(cubeMap);
}
void CubeMapDemo::Update()
{
ImGui::SliderFloat3("LightDirection", direction, -1, +1);
sDirection->SetFloatVector(direction);
static bool bWireFrame = false;
ImGui::Checkbox("WireFrame", &bWireFrame);
quad->Pass(bWireFrame == true ? 1 : 0);
plane->Pass(bWireFrame == true ? 1 : 0);
cube->Pass(bWireFrame == true ? 1 : 0);
for (UINT i = 0; i < 10; i++)
{
cylinder[i]->Pass(bWireFrame == true ? 1 : 0);
sphere[i]->Pass(bWireFrame == true ? 1 : 0);
}
quad->Update();
cube->Update();
plane->Update();
for (UINT i = 0; i < 10; i++)
{
cylinder[i]->Update();
sphere[i]->Update();
}
cubeMap->Update();
}
void CubeMapDemo::Render()
{
for (UINT i = 0; i < 10; i++)
{
cylinder[i]->Render();
sphere[i]->Render();
}
quad->Render();
cube->Render();
plane->Render();
cubeMap->Render();
}
void CubeMapDemo::CreateMesh()
{
quad = new MeshQuad(shader);
quad->DiffuseMap(L"Box.png");
plane = new MeshPlane(shader, 2.5, 2.5);
plane->Scale(12, 1, 12);
plane->DiffuseMap(L"floor.png");
cube = new MeshCube(shader);
cube->Scale(20, 10, 20);
cube->Position(0, 5, 0);
cube->DiffuseMap(L"Stones.png");
for (UINT i = 0; i < 5; i++)
{
//왼쪽
cylinder[i * 2 + 0] = new MeshCylinder(shader, 0.3f, 0.5f, 3.0f, 20, 20);
cylinder[i * 2 + 0]->Position(-30, 6, (float)i * 15.0f - 15.0f);
cylinder[i * 2 + 0]->Scale(5, 5, 5);
cylinder[i * 2 + 0]->DiffuseMap(L"Bricks.png");
//오른쪽
cylinder[i * 2 + 1] = new MeshCylinder(shader, 0.3f, 0.5f, 3.0f, 20, 20);
cylinder[i * 2 + 1]->Position(+30, 6, (float)i * 15.0f - 15.0f);
cylinder[i * 2 + 1]->Scale(5, 5, 5);
cylinder[i * 2 + 1]->DiffuseMap(L"Bricks.png");
//왼쪽
sphere[i * 2 + 0] = new MeshSphere(shader,0.5f, 20, 20);
sphere[i * 2 + 0]->Position(-30, 15.5f, (float)i * 15.0f - 15.0f);
sphere[i * 2 + 0]->Scale(5, 5, 5);
sphere[i * 2 + 0]->DiffuseMap(L"Wall.png");
//오른쪽
sphere[i * 2 + 1] = new MeshSphere(shader, 0.5f, 20, 20);
sphere[i * 2 + 1]->Position(+30, 15.5f, (float)i * 15.0f - 15.0f);
sphere[i * 2 + 1]->Scale(5, 5, 5);
sphere[i * 2 + 1]->DiffuseMap(L"Wall.png");
}
}
#pragma once
#include "Systems/IExecute.h"
class CubeMapDemo : public IExecute
{
public:
virtual void Initialize() override;
virtual void Destroy() override;
virtual void Update() override;
virtual void PreRender() override {};
virtual void Render() override;
virtual void PostRender() override {};
virtual void ResizeScreen() override {};
private:
void CreateMesh();
private:
Shader* shader;
Vector3 direction = Vector3(-1, -1, 1);
ID3DX11EffectVectorVariable* sDirection;
MeshQuad* quad;
MeshPlane* plane;
MeshCube* cube;
MeshCylinder* cylinder[10];
MeshSphere* sphere[10];
Shader* cubeMapShader;
CubeMap* cubeMap;
};
16_CubeMap.fx
더보기
matrix World, View, Projection;
TextureCube CubeMap;
struct VertexInput
{
float4 Position : Position;
};
struct VertexOutput
{
float4 Position : SV_Position;
float3 oPosition : Position1;
};
RasterizerState FillMode_WireFrame{ FillMode = WireFrame; };
SamplerState PointSampler
{
Filter = MIN_MAG_MIP_POINT;
AddressU = Wrap;
AddressV = Wrap;
};
SamplerState LinearSampler
{
Filter = MIN_MAG_MIP_LINEAR;
AddressU = Wrap;
AddressV = Wrap;
};
VertexOutput VS(VertexInput input)
{
VertexOutput output;
output.oPosition = input.Position.xyz;
output.Position = mul(input.Position, World);
output.Position = mul(output.Position, View);
output.Position = mul(output.Position, Projection);
return output;
}
float4 PS(VertexOutput input) : SV_Target
{
return CubeMap.Sample(LinearSampler, input.oPosition);
}
technique11 T0
{
pass P0
{
SetVertexShader(CompileShader(vs_5_0, VS()));
SetPixelShader(CompileShader(ps_5_0, PS()));
}
pass P1
{
SetRasterizerState(FillMode_WireFrame);
SetVertexShader(CompileShader(vs_5_0, VS()));
SetPixelShader(CompileShader(ps_5_0, PS()));
}
}
실행
보충
더보기
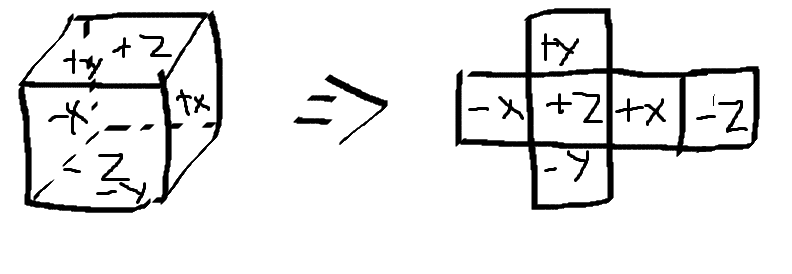
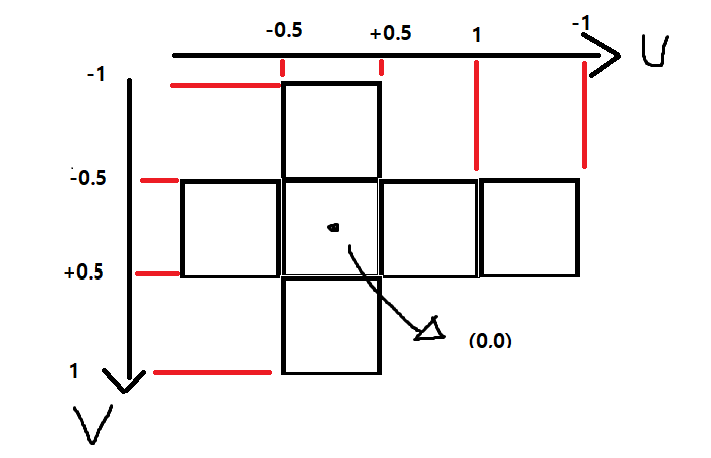
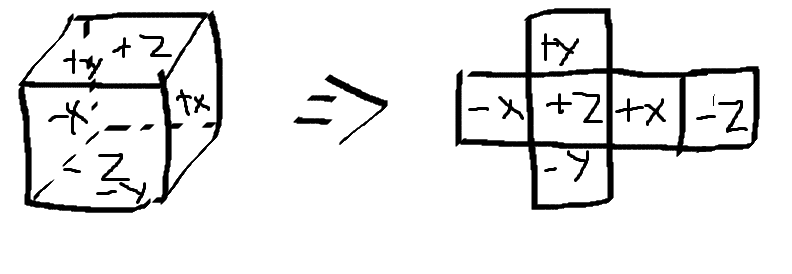
CubeMap은 위에 그림처럼 큐브맵 보는 방식은 저렇고
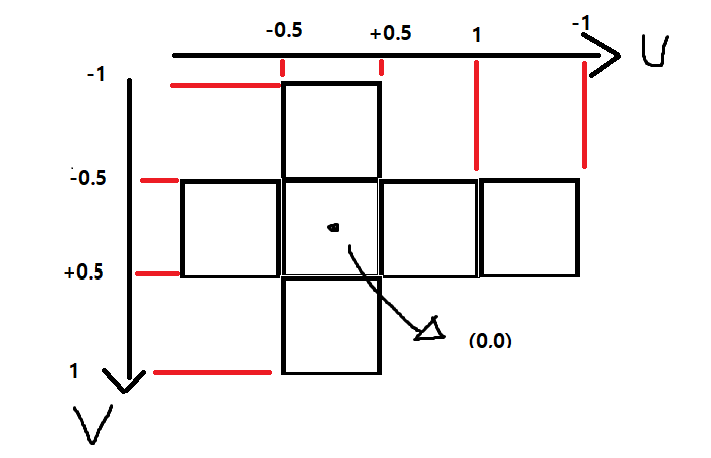
이걸 UV 좌표로 사용하면 Z+ 정가운데 기준으로 0.0 으로 쓸수 있고
UV좌표를 사용 할려면 기존에 Position 위치에 맞게 UV좌표도 비율에 맞게 써야 했다면 CubeMap Texture 같은
경우에는 기존 쓰던 NDC좌표계로 써야 하므로 따로 UV좌표계를 선언해서 사용할 필요가 없다
제목만 수정 하고 CubeMap을 이용해서 sky, 배경을 적용할 예정
'DirectX > DirectX 3D_(구)' 카테고리의 다른 글
17_Framework (Transform, PerFrame, Renderer) (0) | 2021.07.13 |
---|---|
17_Framework (Buffer + hlsl) (0) | 2021.07.07 |
15_Mesh (0) | 2021.07.06 |
13_BaseMap (0) | 2021.07.04 |
12_Normal [1/2] (0) | 2021.07.03 |