728x90
셋팅
더보기
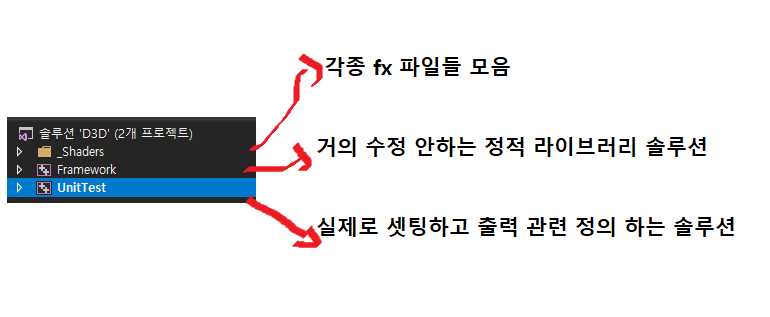
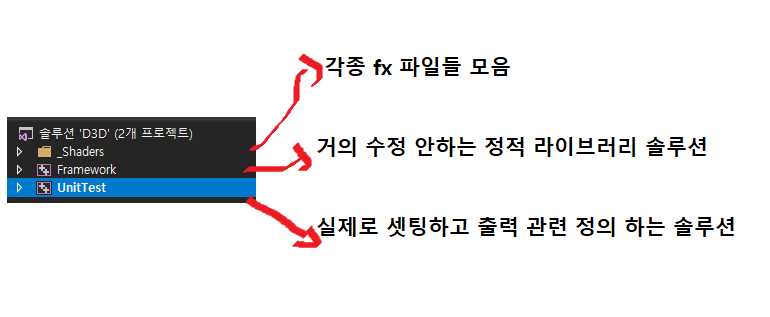
총 3분류로 나뉘어 지고 UnitTest 솔루션에서 실제로 작업해서 출력하는 코드를 작성하고 실행
VertexLine2Demo.h
더보기
#pragma once
#include "Systems/IExecute.h"
class VertexLine2Demo : public IExecute
{
public:
virtual void Initialize() override;
virtual void Destroy() override;
virtual void Update() override;
virtual void PreRender() override {};
virtual void Render() override;
virtual void PostRender() override {};
virtual void ResizeScreen() override {};
private:
Shader* shader;
Vertex vertices[6];
ID3D11Buffer* vertexBuffer;
};
VertexLine2Demo.h.cpp
더보기
#include "stdafx.h"
#include "VertexLine2Demo.h"
void VertexLine2Demo::Initialize()
{
shader = new Shader(L"02_Pass.fx");
vertices[0].Position = Vector3(0, 0, 0);
vertices[1].Position = Vector3(1, 0, 0);
vertices[2].Position = Vector3(0, 0.5, 0);
vertices[3].Position = Vector3(1, 0.5, 0);
vertices[4].Position = Vector3(0, -0.5, 0);
vertices[5].Position = Vector3(1, -0.5, 0);
D3D11_BUFFER_DESC desc;
ZeroMemory(&desc, sizeof(D3D11_BUFFER_DESC));
desc.ByteWidth = sizeof(Vertex) * 6;
desc.BindFlags = D3D11_BIND_VERTEX_BUFFER;
D3D11_SUBRESOURCE_DATA data = { 0 };
data.pSysMem = vertices;
Check(D3D::GetDevice()->CreateBuffer(&desc, &data, &vertexBuffer));
}
void VertexLine2Demo::Destroy()
{
SafeDelete(shader);
SafeRelease(vertexBuffer);
}
void VertexLine2Demo::Update()
{
}
void VertexLine2Demo::Render()
{
UINT stride = sizeof(Vertex);
UINT offset = 0;
D3D::GetDC()->IASetVertexBuffers(0, 1, &vertexBuffer, &stride, &offset);
static bool bStrip = false;
{
ImGui::Checkbox("Strip", &bStrip);
D3D11_PRIMITIVE_TOPOLOGY topology[2] =
{
D3D11_PRIMITIVE_TOPOLOGY_LINELIST,
D3D11_PRIMITIVE_TOPOLOGY_LINESTRIP
};
D3D::GetDC()->IASetPrimitiveTopology(bStrip ? topology[1] : topology[0]);
}
static UINT startLocation = 0;
{
ImGui::SliderInt("Start", (int*)&startLocation, 0 , 6);
}
static UINT pass = 0;
{
ImGui::InputInt("Pass", (int*)&pass);
pass = (UINT)Math::Clamp((int)pass, 0, 3);
static Color color = Color(1, 1, 1, 1);
ImGui::ColorEdit3("Color", color);
shader->AsVector("Color")->SetFloatVector(color);
}
shader->Draw(0, pass, 6, startLocation);
}
Main.cpp
더보기
#include "stdafx.h"
#include "Main.h"
#include "Systems/Window.h"
#include "VertexLineDemo.h"
#include "VertexLineDemoColor.h"
#include "VertexLine2Demo.h"
void Main::Initialize()
{
//Push(new VertexLineDemo());
//Push(new VertexLineDemoColor());
Push(new VertexLine2Demo());
}
void Main::Destroy()
{
for (IExecute* exe : executes)
{
exe->Destroy();
SafeDelete(exe);
}
}
void Main::Update()
{
for (IExecute* exe : executes)
exe->Update();
}
void Main::PreRender()
{
for (IExecute* exe : executes)
exe->PreRender();
}
void Main::Render()
{
for (IExecute* exe : executes)
exe->Render();
}
void Main::PostRender()
{
for (IExecute* exe : executes)
exe->PostRender();
}
void Main::ResizeScreen()
{
for (IExecute* exe : executes)
exe->ResizeScreen();
}
void Main::Push(IExecute * execute)
{
executes.push_back(execute);
execute->Initialize();
}
//윈도우 메인
int WINAPI WinMain(HINSTANCE instance, HINSTANCE prevInstance, LPSTR param, int command)
{
D3DDesc desc;
desc.AppName = L"D3D Game";
desc.Instance = instance;
desc.bFullScreen = false;
desc.bVsync = false;
desc.Handle = NULL;
desc.Width = 1280;
desc.Height = 720;
desc.Background = Color(0.3f, 0.3f, 0.3f, 1.0f);
D3D::SetDesc(desc);
Main* main = new Main();
//랜더링, 업데이트 관련 내용
WPARAM wParam = Window::Run(main);
SafeDelete(main);
return wParam;
}
02_Pass.fx
더보기
struct VertexInput
{
float4 Position : Position;
};
struct VertexOutput
{
float4 Position : SV_Position;
};
VertexOutput VS(VertexInput input)
{
VertexOutput output;
output.Position = input.Position;
return output;
}
float4 PS_Red(VertexOutput input) :SV_Target
{
return float4(1, 0, 0, 1);
}
float4 PS_Green(VertexOutput input) : SV_Target
{
return float4(0, 1, 0, 1);
}
float4 PS_Blue(VertexOutput input) : SV_Target
{
return float4(0, 0, 1, 1);
}
float3 Color;
float4 PS_Value(VertexOutput input) : SV_Target
{
return float4(Color, 1);
}
technique11 T0
{
pass P0
{
SetVertexShader(CompileShader(vs_5_0, VS()));
SetPixelShader(CompileShader(ps_5_0, PS_Red()));
}
pass P1
{
SetVertexShader(CompileShader(vs_5_0, VS()));
SetPixelShader(CompileShader(ps_5_0, PS_Green()));
}
pass P2
{
SetVertexShader(CompileShader(vs_5_0, VS()));
SetPixelShader(CompileShader(ps_5_0, PS_Blue()));
}
pass P3
{
SetVertexShader(CompileShader(vs_5_0, VS()));
SetPixelShader(CompileShader(ps_5_0, PS_Value()));
}
}
//technique11 진행순서
// VS -> RS -> PS
//semantic SV 의미 : System약어 Pixel화 가능함
출력
'DirectX > DirectX 3D_(구)' 카테고리의 다른 글
07_Camera (0) | 2021.06.30 |
---|---|
06_Grid (0) | 2021.06.30 |
05_Index (0) | 2021.06.30 |
04_World (0) | 2021.06.28 |
03_Triangle, Rect (0) | 2021.06.28 |